在使用 Python 的开发过程中,除了使用 datetime 标准库来处理时间和日期,还有许多第三方的开源库值得尝试。
Arrow 是一个专门处理时间和日期的轻量级 Python 库,它提供了一种合理、智能的方式来创建、操作、格式化、转换时间和日期,并提供了一个支持许多常见构建方案的智能模块 API 。简单来说,它可以帮你以更简便的操作和更少的代码来使用日期和时间。其设计灵感主要来源于 moment.js 和 requests 。
Quick start
$ pip install arrow
>>> import arrow >>> utc = arrow.utcnow() >>> utc <Arrow [2013-05-11T21:23:58.970460+00:00]> >>> utc = utc.replace(hours=-1) >>> utc <Arrow [2013-05-11T20:23:58.970460+00:00]> >>> local = utc.to('US/Pacific') >>> local <Arrow [2013-05-11T13:23:58.970460-07:00]> >>> arrow.get('2013-05-11T21:23:58.970460+00:00') <Arrow [2013-05-11T21:23:58.970460+00:00]> >>> local.timestamp 1368303838 >>> local.format() '2013-05-11 13:23:58 -07:00' >>> local.format('YYYY-MM-DD HH:mm:ss ZZ') '2013-05-11 13:23:58 -07:00' >>> local.humanize() 'an hour ago' >>> local.humanize(locale='ko_kr') '1시간 전'
Delorean 提供了一个相比于 datetime 和 pytz 的更好的抽象,让你处理时间更容易。它有很多有用的处理时区的特性,标准化时区或者从一个时区改变到另外一个时区。
Quick start
from datetime import datetime import pytz est = pytz.timezone('US/Eastern') d = datetime.now(pytz.utc) d = est.normalize(d.astimezone(est)) return d
from delorean import Delorean d = Delorean() d = d.shift('US/Eastern') return d
原生的 datetime 足够应付基本情况,但当面对更复杂的用例时,通常会有的捉襟见肘,不那么直观。 Pendulum 在标准库的基础之上,提供了一个更简洁,更易于使用的 API ,旨在让 Python datetime 更好用。
Quick start
>>> import pendulum >>> now_in_paris = pendulum.now('Europe/Paris') >>> now_in_paris '2016-07-04T00:49:58.502116+02:00' # Seamless timezone switching >>> now_in_paris.in_timezone('UTC') '2016-07-03T22:49:58.502116+00:00' >>> tomorrow = pendulum.now().add(days=1) >>> last_week = pendulum.now().subtract(weeks=1) >>> if pendulum.now().is_weekend(): ... print('Party!') 'Party!' >>> past = pendulum.now().subtract(minutes=2) >>> past.diff_for_humans() >>> '2 minutes ago' >>> delta = past - last_week >>> delta.hours 23 >>> delta.in_words(locale='en') '6 days 23 hours 58 minutes' # Proper handling of datetime normalization >>> pendulum.create(2013, 3, 31, 2, 30, 0, 0, 'Europe/Paris') '2013-03-31T03:30:00+02:00' # 2:30 does not exist (Skipped time) # Proper handling of dst transitions >>> just_before = pendulum.create(2013, 3, 31, 1, 59, 59, 999999, 'Europe/Paris') '2013-03-31T01:59:59.999999+01:00' >>> just_before.add(microseconds=1) '2013-03-31T03:00:00+02:00'
dateutil 是 datetime 标准库的一个扩展库,几乎支持以所有字符串格式对日期进行通用解析,日期计算灵活,内部数据更新及时。
Quick start
>>> from dateutil.relativedelta import * >>> from dateutil.easter import * >>> from dateutil.rrule import * >>> from dateutil.parser import * >>> from datetime import * >>> now = parse("Sat Oct 11 17:13:46 UTC 2003") >>> today = now.date() >>> year = rrule(YEARLY,dtstart=now,bymonth=8,bymonthday=13,byweekday=FR)[0].year >>> rdelta = relativedelta(easter(year), today) >>> print("Today is: %s" % today) Today is: 2003-10-11 >>> print("Year with next Aug 13th on a Friday is: %s" % year) Year with next Aug 13th on a Friday is: 2004 >>> print("How far is the Easter of that year: %s" % rdelta) How far is the Easter of that year: relativedelta(months=+6) >>> print("And the Easter of that year is: %s" % (today+rdelta)) And the Easter of that year is: 2004-04-11
用于处理日期/时间的 Python 库,设计灵感同样是来源于 moment.js 和 requests ,设计理念源自 Times Python 模块。
Usage
import moment from datetime import datetime # Create a moment from a string moment.date("12-18-2012") # Create a moment with a specified strftime format moment.date("12-18-2012", "%m-%d-%Y") # Moment uses the awesome dateparser library behind the scenes moment.date("2012-12-18") # Create a moment with words in it moment.date("December 18, 2012") # Create a moment that would normally be pretty hard to do moment.date("2 weeks ago") # Create a future moment that would otherwise be really difficult moment.date("2 weeks from now") # Create a moment from the current datetime moment.now() # The moment can also be UTC-based moment.utcnow() # Create a moment with the UTC time zone moment.utc("2012-12-18") # Create a moment from a Unix timestamp moment.unix(1355875153626) # Create a moment from a Unix UTC timestamp moment.unix(1355875153626, utc=True) # Return a datetime instance moment.date(2012, 12, 18).date # We can do the same thing with the UTC method moment.utc(2012, 12, 18).date # Create and format a moment using Moment.js semantics moment.now().format("YYYY-M-D") # Create and format a moment with strftime semantics moment.date(2012, 12, 18).strftime("%Y-%m-%d") # Update your moment's time zone moment.date(datetime(2012, 12, 18)).locale("US/Central").date # Alter the moment's UTC time zone to a different time zone moment.utcnow().timezone("US/Eastern").date # Set and update your moment's time zone. For instance, I'm on the # west coast, but want NYC's current time. moment.now().locale("US/Pacific").timezone("US/Eastern") # In order to manipulate time zones, a locale must always be set or # you must be using UTC. moment.utcnow().timezone("US/Eastern").date # You can also clone a moment, so the original stays unaltered now = moment.utcnow().timezone("US/Pacific") future = now.clone().add(weeks=2)
提供对用户非常友好的特性来帮助执行常见的日期和时间操作。
Usage
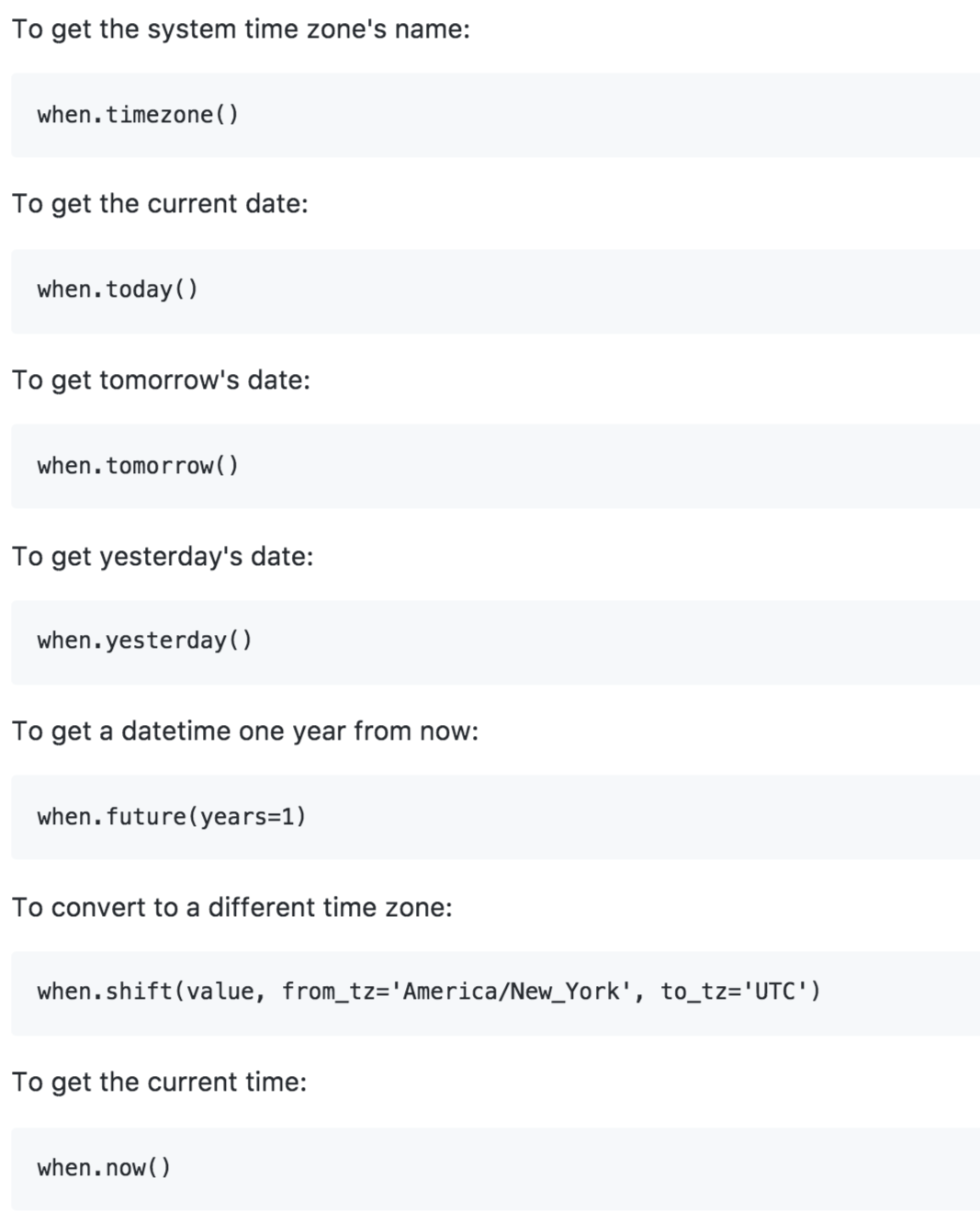