注:以下分析均基于Guava18
背景
除了我们会如此命名XXXService2 原来Google也会这样命名O(∩_∩)O哈!
由于jdk已经占据了Collections类名 因此Google在Collection基础上创建了
Collections2
基本知识
Google的工具类通常以s结尾 比如Lists Sets Collections2 Ints Strings 等等
进阶
filter
相对于Lists来说filter可以对易到transform方法,提供了一个过滤集合元素的方法
这也是开发过程中常见的用法,给定一个集合 通过某种方式过滤掉一些元素
/** * Returns the elements of {@code unfiltered} that satisfy a predicate. The * returned collection is a live view of {@code unfiltered}; changes to one * affect the other. * * <p>The resulting collection's iterator does not support {@code remove()}, * but all other collection methods are supported. When given an element that * doesn't satisfy the predicate, the collection's {@code add()} and {@code * addAll()} methods throw an {@link IllegalArgumentException}. When methods * such as {@code removeAll()} and {@code clear()} are called on the filtered * collection, only elements that satisfy the filter will be removed from the * underlying collection. * * <p>The returned collection isn't threadsafe or serializable, even if * {@code unfiltered} is. * * <p>Many of the filtered collection's methods, such as {@code size()}, * iterate across every element in the underlying collection and determine * which elements satisfy the filter. When a live view is <i>not</i> needed, * it may be faster to copy {@code Iterables.filter(unfiltered, predicate)} * and use the copy. * * <p><b>Warning:</b> {@code predicate} must be <i>consistent with equals</i>, * as documented at {@link Predicate#apply}. Do not provide a predicate such * as {@code Predicates.instanceOf(ArrayList.class)}, which is inconsistent * with equals. (See {@link Iterables#filter(Iterable, Class)} for related * functionality.) */ // TODO(kevinb): how can we omit that Iterables link when building gwt // javadoc? public static <E> Collection<E> filter( Collection<E> unfiltered, Predicate<? super E> predicate) { if (unfiltered instanceof FilteredCollection) { // Support clear(), removeAll(), and retainAll() when filtering a filtered // collection. return ((FilteredCollection<E>) unfiltered).createCombined(predicate); } return new FilteredCollection<E>( checkNotNull(unfiltered), checkNotNull(predicate)); }
和之前Lists的相关方法类似 Guava的处理都是通过抽象出一个函数 将函数传入,在对应多个方法之前进行处理
那么首先看一下Predicate接口
/** * Determines a true or false value for a given input. * * <p>The {@link Predicates} class provides common predicates and related utilities. * * <p>See the Guava User Guide article on <a href= * "http://code.google.com/p/guava-libraries/wiki/FunctionalExplained">the use of {@code * Predicate}</a>. * * @author Kevin Bourrillion * @since 2.0 (imported from Google Collections Library) */ @GwtCompatible public interface Predicate<T> { /** * Returns the result of applying this predicate to {@code input}. This method is <i>generally * expected</i>, but not absolutely required, to have the following properties: * * <ul> * <li>Its execution does not cause any observable side effects. * <li>The computation is <i>consistent with equals</i>; that is, {@link Objects#equal * Objects.equal}{@code (a, b)} implies that {@code predicate.apply(a) == * predicate.apply(b))}. * </ul> * * @throws NullPointerException if {@code input} is null and this predicate does not accept null * arguments */ boolean apply(@Nullable T input); /** * Indicates whether another object is equal to this predicate. * * <p>Most implementations will have no reason to override the behavior of {@link Object#equals}. * However, an implementation may also choose to return {@code true} whenever {@code object} is a * {@link Predicate} that it considers <i>interchangeable</i> with this one. "Interchangeable" * <i>typically</i> means that {@code this.apply(t) == that.apply(t)} for all {@code t} of type * {@code T}). Note that a {@code false} result from this method does not imply that the * predicates are known <i>not</i> to be interchangeable. */ @Override boolean equals(@Nullable Object object); }
方法apply需要一个返回值 true表示该元素需要保留 false则需要在新的集合不保留
正如上文所说Guava的工具类通常以s结尾
因此Guava也提供了一些常见的Predicate 可以通过Predicates获取
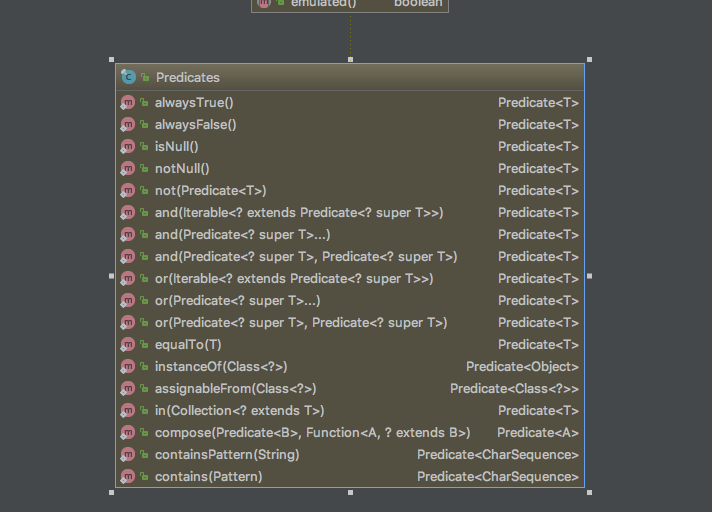
一般来说我们会使用notNull等 其也提供了 and or等语法 甚至正则等等 十分强大。即使仍然不然满足条件还是可以自定义
可以看到了这边存在一个小问题 Lists没有filter方法 因此想要获得list还得需要Lists.newArrayList
考虑一下为何Lists没有对应的filter方法 可以参考 guava-why-is-there-no-lists-filter-function
It wasn't implemented because it would expose a perilous large number of slow methods, such as #get(index) on the returned List view (inviting performance bugs). And ListIterator would be a pain to implement as well (though I submitted a patch years ago to cover that).
Since indexed methods can't be efficient in the filtered List view, it's better to just go with a filtered Iterable, which doesn't have them
orderedPermutations
排列也是我们常使用的功能
/** * Returns a {@link Collection} of all the permutations of the specified * {@link Iterable}. * * <p><i>Notes:</i> This is an implementation of the algorithm for * Lexicographical Permutations Generation, described in Knuth's "The Art of * Computer Programming", Volume 4, Chapter 7, Section 7.2.1.2. The * iteration order follows the lexicographical order. This means that * the first permutation will be in ascending order, and the last will be in * descending order. * * <p>Duplicate elements are considered equal. For example, the list [1, 1] * will have only one permutation, instead of two. This is why the elements * have to implement {@link Comparable}. * * <p>An empty iterable has only one permutation, which is an empty list. * * <p>This method is equivalent to * {@code Collections2.orderedPermutations(list, Ordering.natural())}. * * @param elements the original iterable whose elements have to be permuted. * @return an immutable {@link Collection} containing all the different * permutations of the original iterable. * @throws NullPointerException if the specified iterable is null or has any * null elements. * @since 12.0 */ @Beta public static <E extends Comparable<? super E>> Collection<List<E>> orderedPermutations(Iterable<E> elements) { return orderedPermutations(elements, Ordering.natural()); } /** * Returns a {@link Collection} of all the permutations of the specified * {@link Iterable} using the specified {@link Comparator} for establishing * the lexicographical ordering. * * <p>Examples: <pre> {@code * * for (List<String> perm : orderedPermutations(asList("b", "c", "a"))) { * println(perm); * } * // -> ["a", "b", "c"] * // -> ["a", "c", "b"] * // -> ["b", "a", "c"] * // -> ["b", "c", "a"] * // -> ["c", "a", "b"] * // -> ["c", "b", "a"] * * for (List<Integer> perm : orderedPermutations(asList(1, 2, 2, 1))) { * println(perm); * } * // -> [1, 1, 2, 2] * // -> [1, 2, 1, 2] * // -> [1, 2, 2, 1] * // -> [2, 1, 1, 2] * // -> [2, 1, 2, 1] * // -> [2, 2, 1, 1]}</pre> * * <p><i>Notes:</i> This is an implementation of the algorithm for * Lexicographical Permutations Generation, described in Knuth's "The Art of * Computer Programming", Volume 4, Chapter 7, Section 7.2.1.2. The * iteration order follows the lexicographical order. This means that * the first permutation will be in ascending order, and the last will be in * descending order. * * <p>Elements that compare equal are considered equal and no new permutations * are created by swapping them. * * <p>An empty iterable has only one permutation, which is an empty list. * * @param elements the original iterable whose elements have to be permuted. * @param comparator a comparator for the iterable's elements. * @return an immutable {@link Collection} containing all the different * permutations of the original iterable. * @throws NullPointerException If the specified iterable is null, has any * null elements, or if the specified comparator is null. * @since 12.0 */ @Beta public static <E> Collection<List<E>> orderedPermutations( Iterable<E> elements, Comparator<? super E> comparator) { return new OrderedPermutationCollection<E>(elements, comparator); }
当只传一个参数 默认是自然序 注释也比较明确了
当然Collections2也有transform方法 和Lists类似