前言:
最近跟我的一个同事在讨论关于---有没有必要重复造轮子? 就我感受而言不重复造轮子让更多的时间去研究更广阔的领域。但前提是你有一定基础,什么基础呢?知道这个轮子它的实现原理是什么?让你独自去实现同样的效果你有把握吗?如果你有把握,那么恭喜你,你完全可以去复制别人的轮子,把时间花费在你未知的领域。当然像比较庞大的第三方,不建议太深入以免脱不了坑,适当就行。
圆扩散:
项目中涉及到一个圆形不断的扩散效果,找了下网上也有类似的效果,但是呢,像扩散速度、间距、屏幕适配等等不是很完善,同时借鉴了代码,自己根据需求完善了这个自定义view。就当做练练手,以后关于这类的题材也会继续更新。
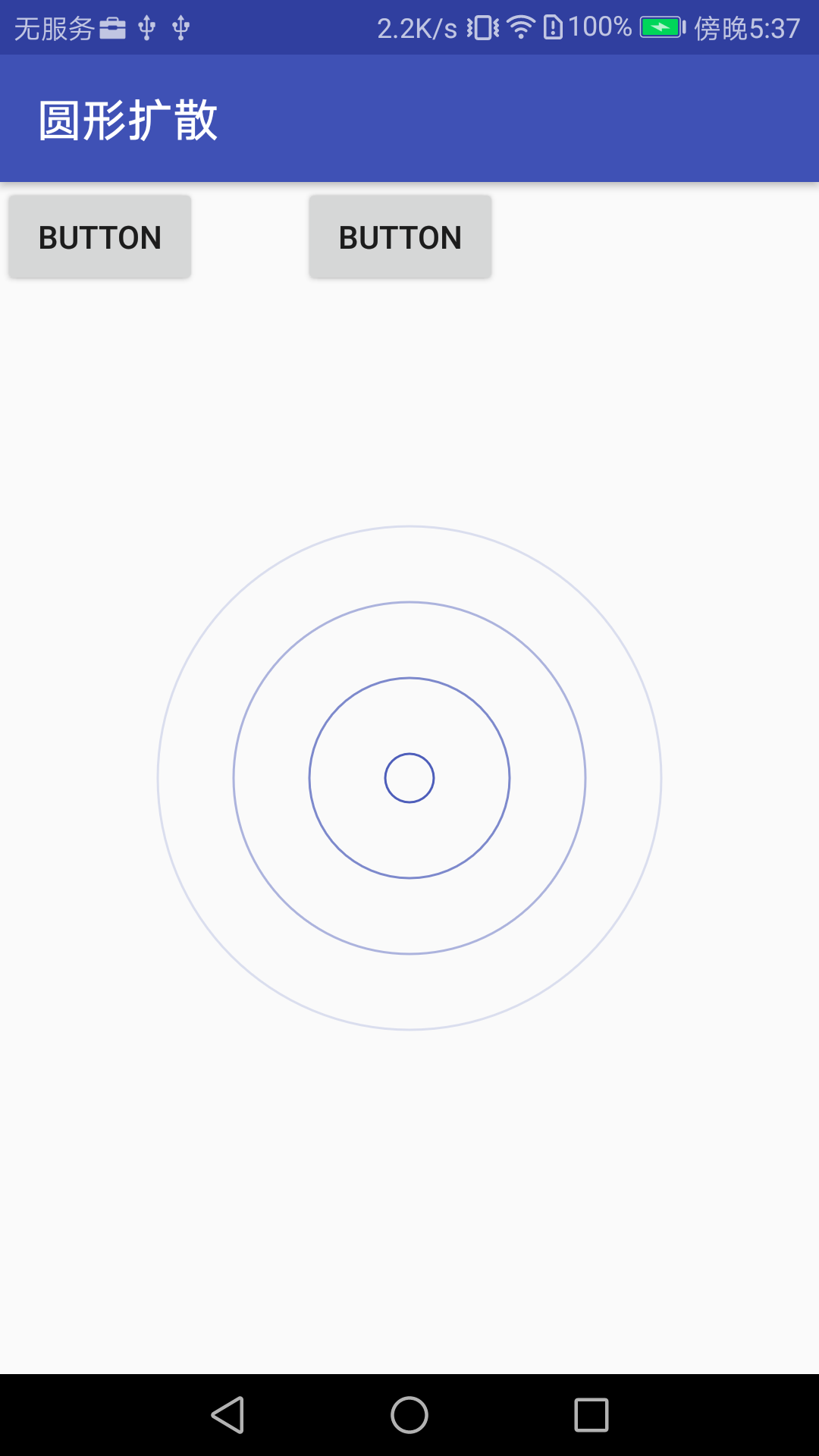
实现步骤:
1.画圆,根据圆的直径不断改变实现扩散
2.添加圆时机, 采用数组遍历画多个圆
3.处理数组中数据。
自定义属性:
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="WaveView"> <attr name="wavecolor" format="color"/> <!--view颜色--> <attr name="fillstyle" format="boolean"/><!--Paint的填充风格--> <attr name="space" format="integer"/><!--每个圆形间距--> <attr name="width" format="integer"/><!--扩散的宽度--> </declare-styleable> </resources>
这个没啥好说的了,name的取名最好和类一致
java代码:
package partyhistorymuseum.lixiaoqian.com.custom_1; import android.content.Context; import android.content.res.TypedArray; import android.graphics.Canvas; import android.graphics.Paint; import android.support.annotation.Nullable; import android.util.AttributeSet; import android.view.View; import java.util.ArrayList; import java.util.List; /** * Created by huangzhibo on 2017/9/25/025. * 步骤:拆分式实现 * 1.先画个圆,通过属性动画扩散(根据圆的radius) * 2.通过255f/view宽*当前drawCiry 去设置透明度 * 3.间隔多久(间距)在画一个圆 * mail:1043202454@qq.com */ public class WaveView extends View { private static int centerColor; private Paint mPaint; private float alpharate; private boolean isStartAnim = false; private int mSpace; private int mWidth; private boolean isFillstyle; private List<Float> widths = new ArrayList(); private List<Integer> mAlphas = new ArrayList(); public WaveView(Context context) { this(context, null); } public WaveView(Context context, @Nullable AttributeSet attrs) { this(context, attrs, 0); } public WaveView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.WaveView, defStyleAttr, 0); centerColor = typedArray.getColor(R.styleable.WaveView_wavecolor, getResources().getColor(R.color.colorAccent)); mSpace = typedArray.getInteger(R.styleable.WaveView_space, 100); isFillstyle = typedArray.getBoolean(R.styleable.WaveView_fillstyle, true); mWidth = typedArray.getInteger(R.styleable.WaveView_width, 400); typedArray.recycle(); init(); } private void init() { mPaint = new Paint(); mPaint.setAntiAlias(true); if (isFillstyle) { mPaint.setStyle(Paint.Style.FILL); } else { mPaint.setStyle(Paint.Style.STROKE); mPaint.setStrokeWidth(3); } alpharate = 255f / mWidth; //注意这里 如果为int类型就会为0,除数中f一定要加,默认int ; mAlphas.add(255); widths.add(0f); } @Override protected void onDraw(Canvas canvas) { if (isStartAnim) { invalidate(); } mPaint.setColor(centerColor); for (int i = 0; i < widths.size(); i++) { //遍历圆数目 Integer cuAlpha = mAlphas.get(i); mPaint.setAlpha(cuAlpha); Float aFloat = widths.get(i); canvas.drawCircle(getWidth() / 2, getHeight() / 2, aFloat, mPaint); //画圆 if (aFloat < mWidth) { //扩散直径和透明度 mAlphas.set(i, (int) (255 - alpharate * aFloat)); widths.set(i, aFloat + 1); } } if (widths.size() >= 5) { mAlphas.remove(0); widths.remove(0); } if (widths.get(widths.size() - 1) == mSpace) { mAlphas.add(255); widths.add(0f); } } public void startAnim() { isStartAnim = true; invalidate(); } public void pauseAnim() { isStartAnim = false; } }
xml布局:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:zhibo="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="partyhistorymuseum.lixiaoqian.com.custom_1.MainActivity"> <partyhistorymuseum.lixiaoqian.com.custom_1.WaveView android:id="@+id/waveView" android:layout_width="400dp" android:layout_height="400dp" zhibo:wavecolor="@color/colorPrimary" zhibo:space="100" zhibo:width="400" zhibo:fillstyle="false" android:layout_centerInParent="true" /> </RelativeLayout>
这里要提几个点:
- alpharate的值是float类型的,应该如果采用int类型在mWidth大于255的时候永远都是等于0,int类型对于余数都是直接舍去取整。
- 对于以下的代码建议放着onDraw最前面,为什么呢?考虑到切换activity时要立马暂停扩散动画。如果不这样做,会有延迟,切换activity会有卡顿视觉差
if (isStartAnim) { invalidate(); }
- 在onDraw中最好不要new对象,在这个view中 是通过不断的重绘实现动画效果。
对于自定义view的特效还有很多,这里有一篇我之前写的高级自定义View仿TextView:https://my.oschina.net/huangzhi1bo/blog/1517689