1.实例一:
题目:输入一行字符串,分别统计出其中英文字母、空格、数字和其它字符的个数。
1.程序分析:利用while语句,条件为输入的字符不为'\n'. #用isdigit函数判断是否数字 #用isalpha判断是否字母 #isalnum判断是否数字和字母的组合 。
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Time : 2018/4/10 22:13 # @Author : Feng Xiaoqing # @File : string.py # @Function: ----------- while 1: strings = input("Please input a string(quit will be exit):") alpha, dig, space, other = 0, 0, 0, 0 if strings.strip() == "quit": exit(1) for i in strings: if i.isdigit(): dig += 1 elif i.isspace(): space += 1 elif i.isalpha(): alpha += 1 else: other += 1 print("alpha = {0}".format(alpha)) print("dig = {0}".format(dig)) print("space = {0}".format(space)) print("other = {0}".format(other))
运行结果:
Please input a string(quit will be exit):abcdefghijklmn1234567890 355kaldsfadsf;la#@@@ alpha = 26 dig = 13 space = 1 other = 5 Please input a string(quit will be exit):
2.实例二:
题目:写出代码实现输入一个数字,可以自动计算这个数的阶乘。
亦即n!=1×2×3×...×n。阶乘亦可以递归方式定义:0!=1,n!=(n-1)!×n。
如:
0! = 1
1! = 1
2! = 2*1=2
3! = 3*2*1=6
程序代码:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Time : 2018/4/10 21:59 # @Author : Feng Xiaoqing # @File : jiecheng_for_n.py # @Function: ----------- def jc(n): result = 1 if n == 0: return result else: for i in range(1,n+1): result *= i return result n = input("Please input number n:") count = 0 for i in range(0,int(n)+1): count += jc(i) print("count = {0}".format(count))
运算结果:
Please input number n:8 count = 46234
3.实例三:
题目:ABCD乘9=DCBA,A=? B=? C=? D=? 计算A、B、C、D的值分别为多少?
答案:a=1,b=0,c=8,d=9 1089*9=9801
程序代码:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Time : 2018/4/10 21:54 # @Author : Feng Xiaoqing # @File : ABCDDCBA.py # @Function: ----------- for A in [1]: for B in range(0,10): for C in range(0,10): for D in [9]: if ((1000*A + 100*B +10*C + D)*9 == 1000*D + 100*C + 10*B + A ): print("A = {0}".format(A)) print("B = {0}".format(B)) print("C = {0}".format(C)) print("D = {0}".format(D)) print("{0}{1}{2}{3}x9={3}{2}{1}{0}".format(A,B,C,D))
运行结果:
A = 1 B = 0 C = 8 D = 9 1089x9=9801
4.实例四:
题目:写出代码实现九宫格数独的计算。
-------------
| A | B | C |
| D | E | F |
| G | H | I |
-------------
A,B,C,D,E,F,G,H,I 取值为1-9
所有的横竖斜线加起来都等于15:
(A+B+C)=(D+E+F)=(G+H+I)=(A+D+G)=(B+E+H)=(C+F+I)=(A+E+I)=(G+E+C)= 15
程序代码:
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Time : 2018/4/10 23:31 # @Author : Feng Xiaoqing # @File : jiu_Gong_Ge.py # @Function: ----------- number = list() for i in range(1, 10): number.append(i) print(number) count = 1 for A in number: a = number.copy() a.remove(A) for B in a: b = a.copy() b.remove(B) for C in b: c = b.copy() c.remove(C) for D in c: d = c.copy() d.remove(D) for E in d: e = d.copy() e.remove(E) for F in e: f = e.copy() f.remove(F) for G in f: g = f.copy() g.remove(G) for H in g: h = g.copy() h.remove(H) for I in h: if (A+B+C == D+E+F == G+H+I == A+D+G == B+E+H == C+F+I == A+E+I == G+E+C == 15): print(''' 第{9}种例子 ------------- | {0} | {1} | {2} | | {3} | {4} | {5} | | {6} | {7} | {8} | -------------'''.format(A,B,C,D,E,F,G,H,I,count)) count += 1
运行结果:
[1, 2, 3, 4, 5, 6, 7, 8, 9] 第1种例子 ------------- | 2 | 7 | 6 | | 9 | 5 | 1 | | 4 | 3 | 8 | ------------- 第2种例子 ------------- | 2 | 9 | 4 | | 7 | 5 | 3 | | 6 | 1 | 8 | ------------- 第3种例子 ------------- | 4 | 3 | 8 | | 9 | 5 | 1 | | 2 | 7 | 6 | ------------- 第4种例子 ------------- | 4 | 9 | 2 | | 3 | 5 | 7 | | 8 | 1 | 6 | ------------- 第5种例子 ------------- | 6 | 1 | 8 | | 7 | 5 | 3 | | 2 | 9 | 4 | ------------- 第6种例子 ------------- | 6 | 7 | 2 | | 1 | 5 | 9 | | 8 | 3 | 4 | ------------- 第7种例子 ------------- | 8 | 1 | 6 | | 3 | 5 | 7 | | 4 | 9 | 2 | ------------- 第8种例子 ------------- | 8 | 3 | 4 | | 1 | 5 | 9 | | 6 | 7 | 2 | -------------
python中的编码问题:
1.utf-8 Pycharm程序中和cmd中都不会出现乱码。
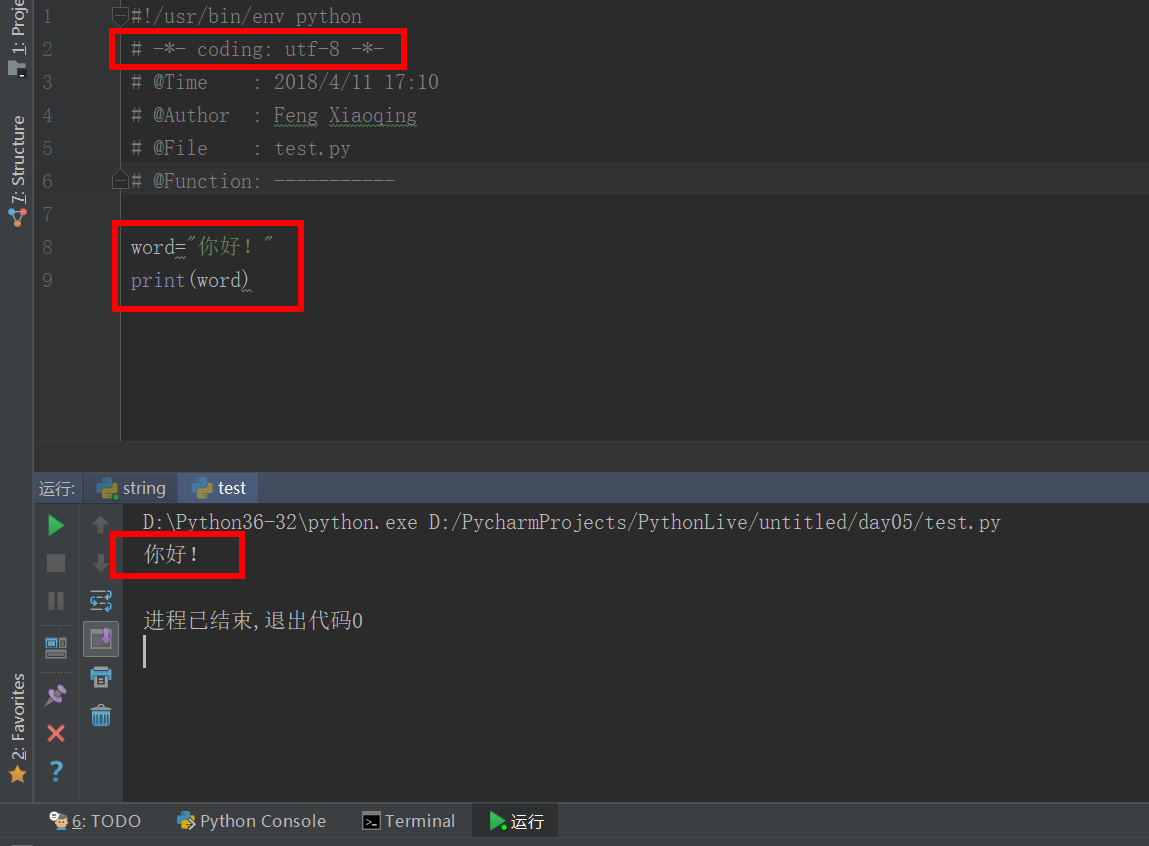
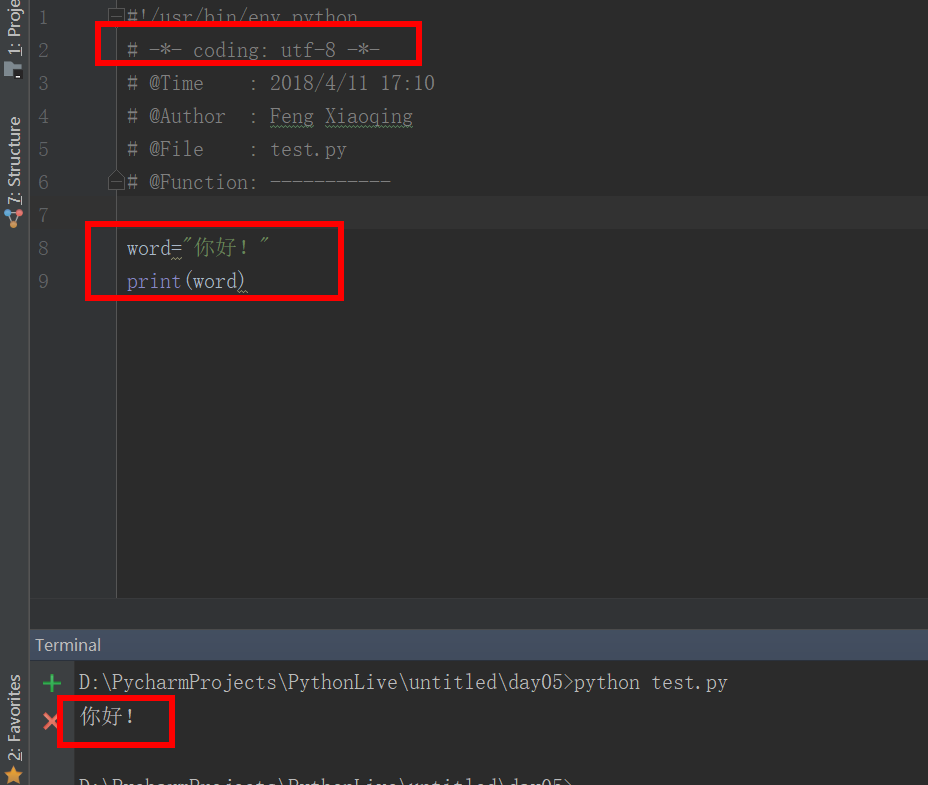
2.当为gbk时都有没乱码
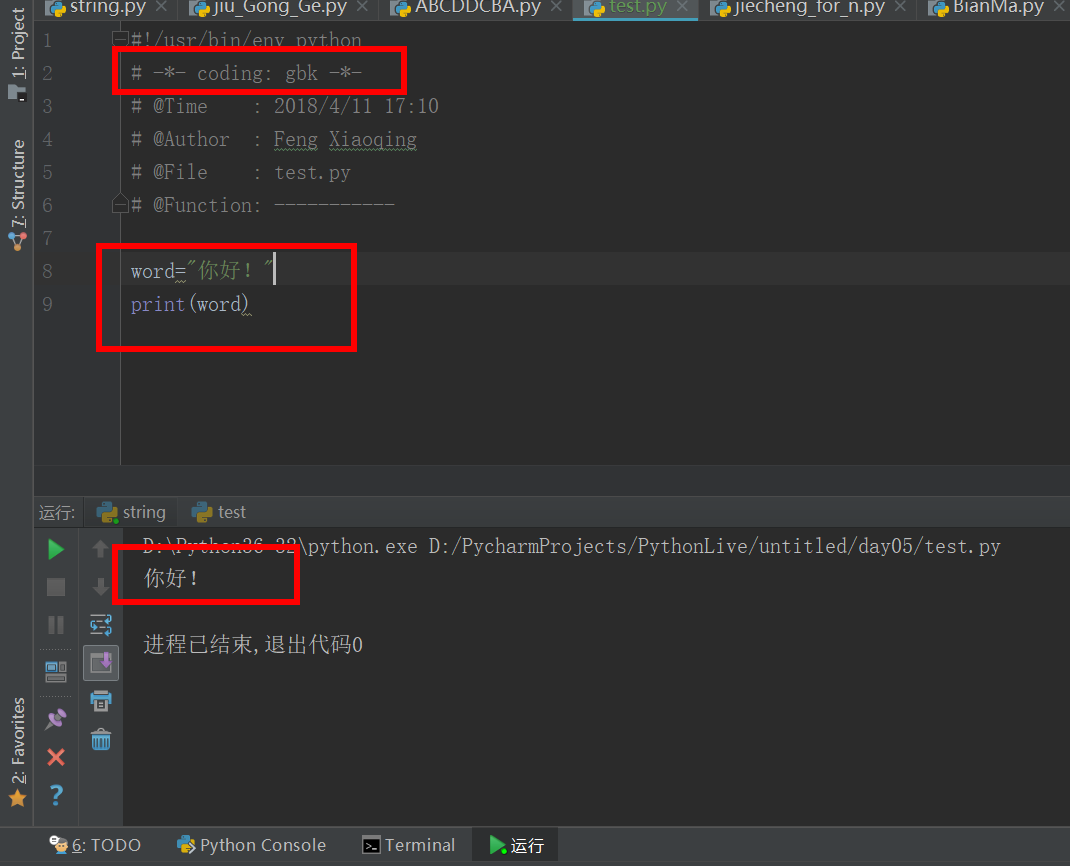
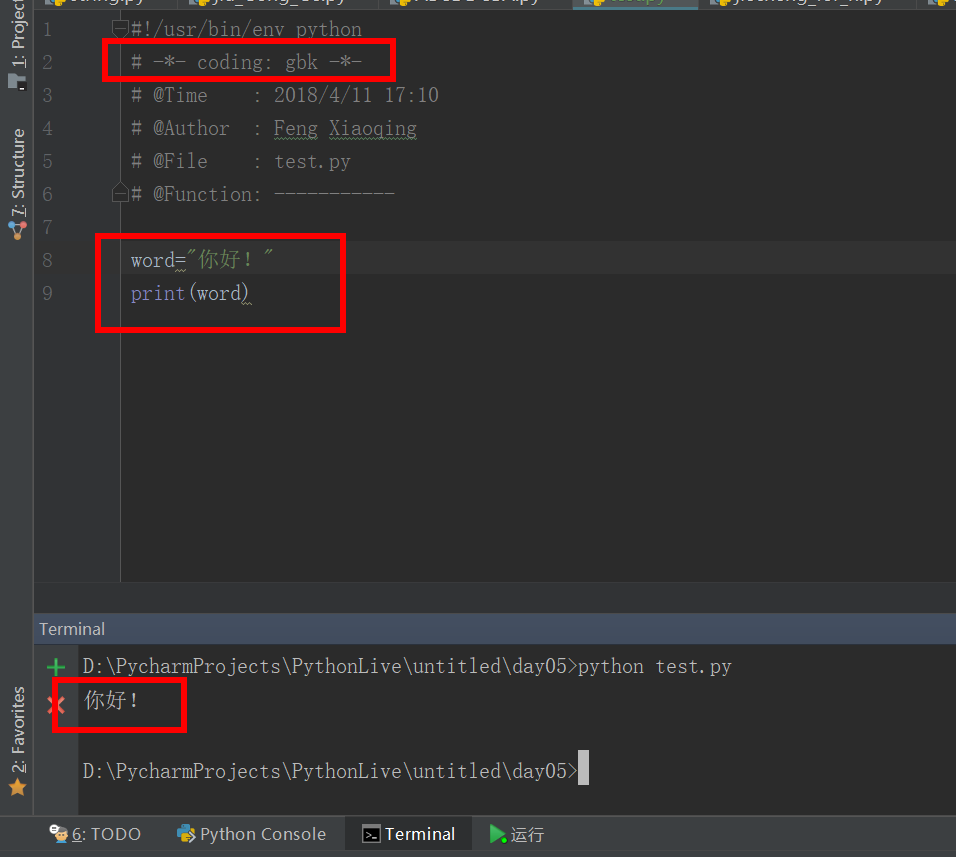
3、文件申明是utf-8的编码,识别“你好”以后,以unicode对象的形式存在。如果我们用type查看,存储形式是unicode,python在向控制台输出unicode对象的时候会自动根据输出环境的编码进行转换。如果输出的不是unicode对象而是str类型。则会按照字符串的编码输出字符串。从而出现utf8没法在gbk编码的控制台展现
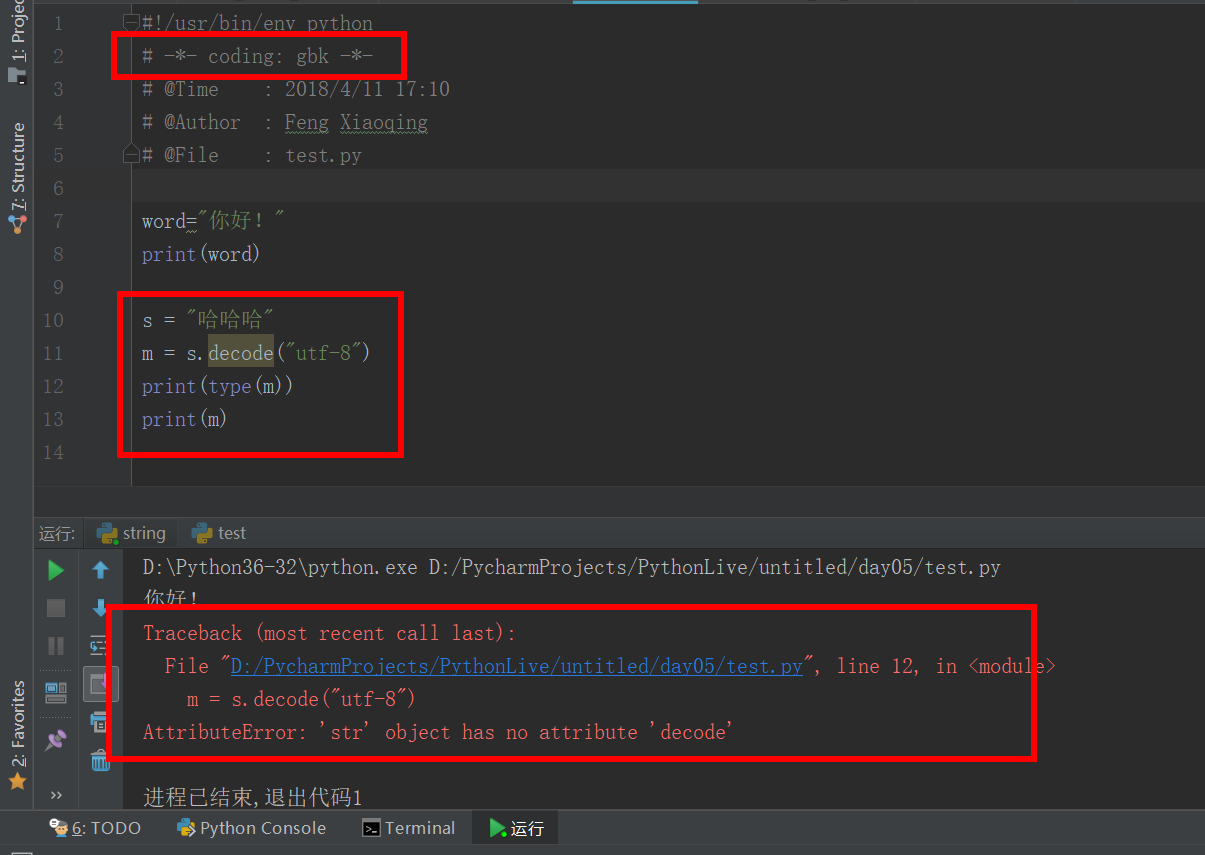
4、列表中Python2中控制台出现乱码,cmd中不会出现乱码,python3中都无乱码:
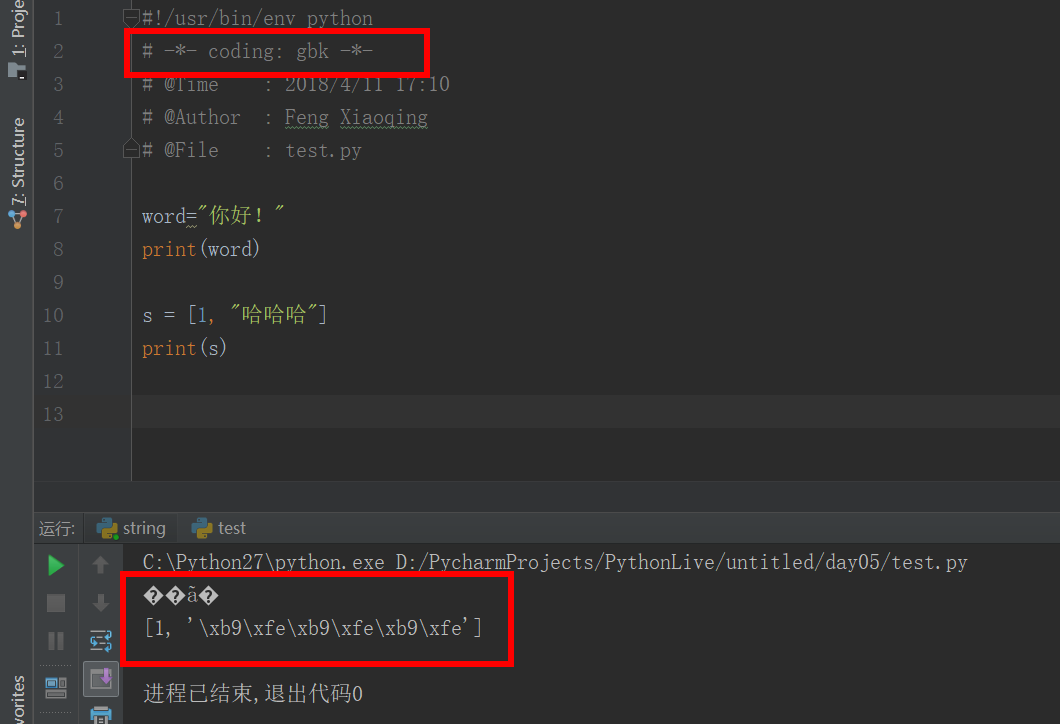
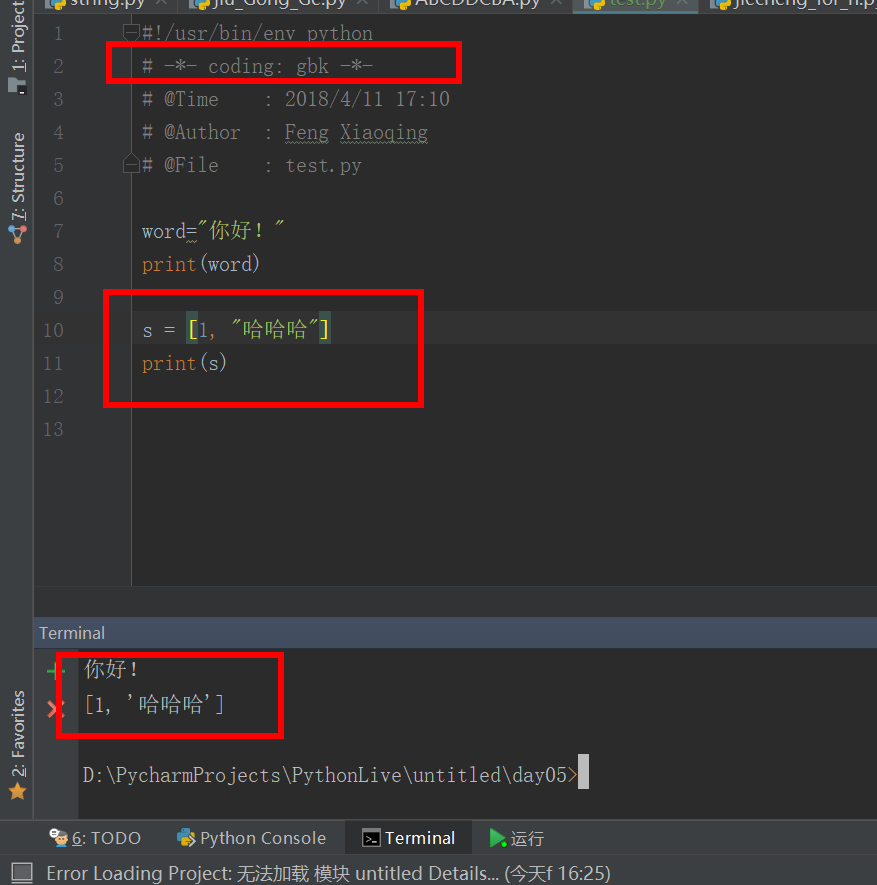
python3中:
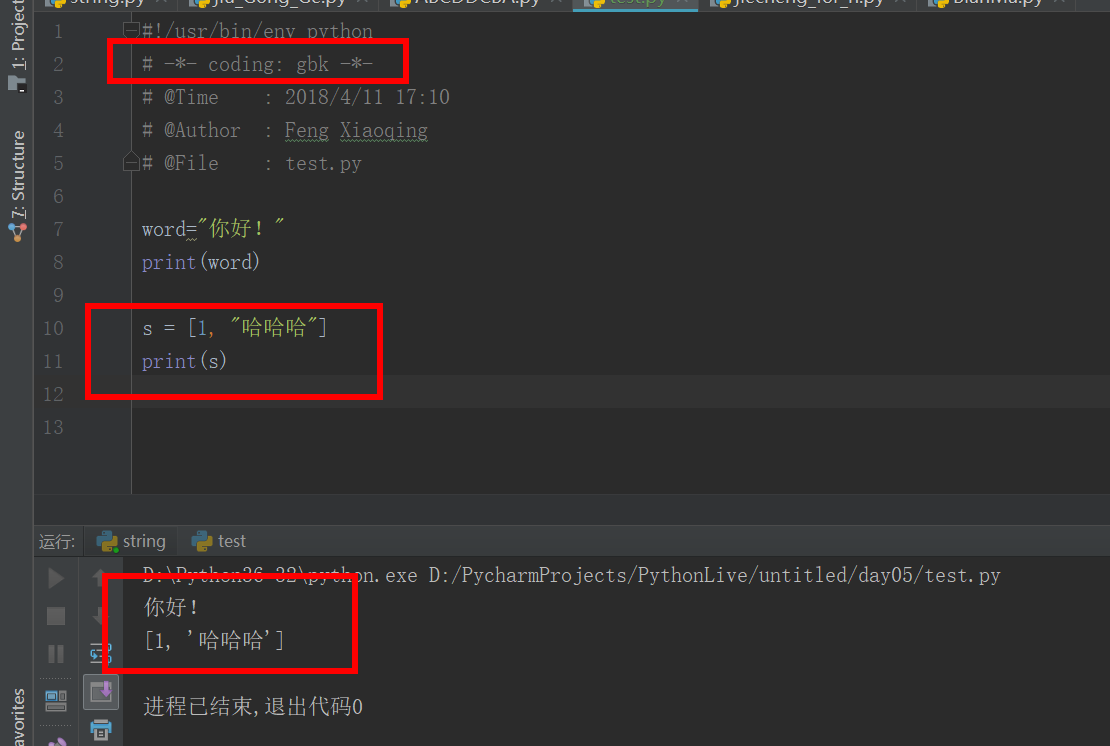
5、编码转换报错:python2中有控制台有乱码,cmd中没有乱码,python3中均无乱码:
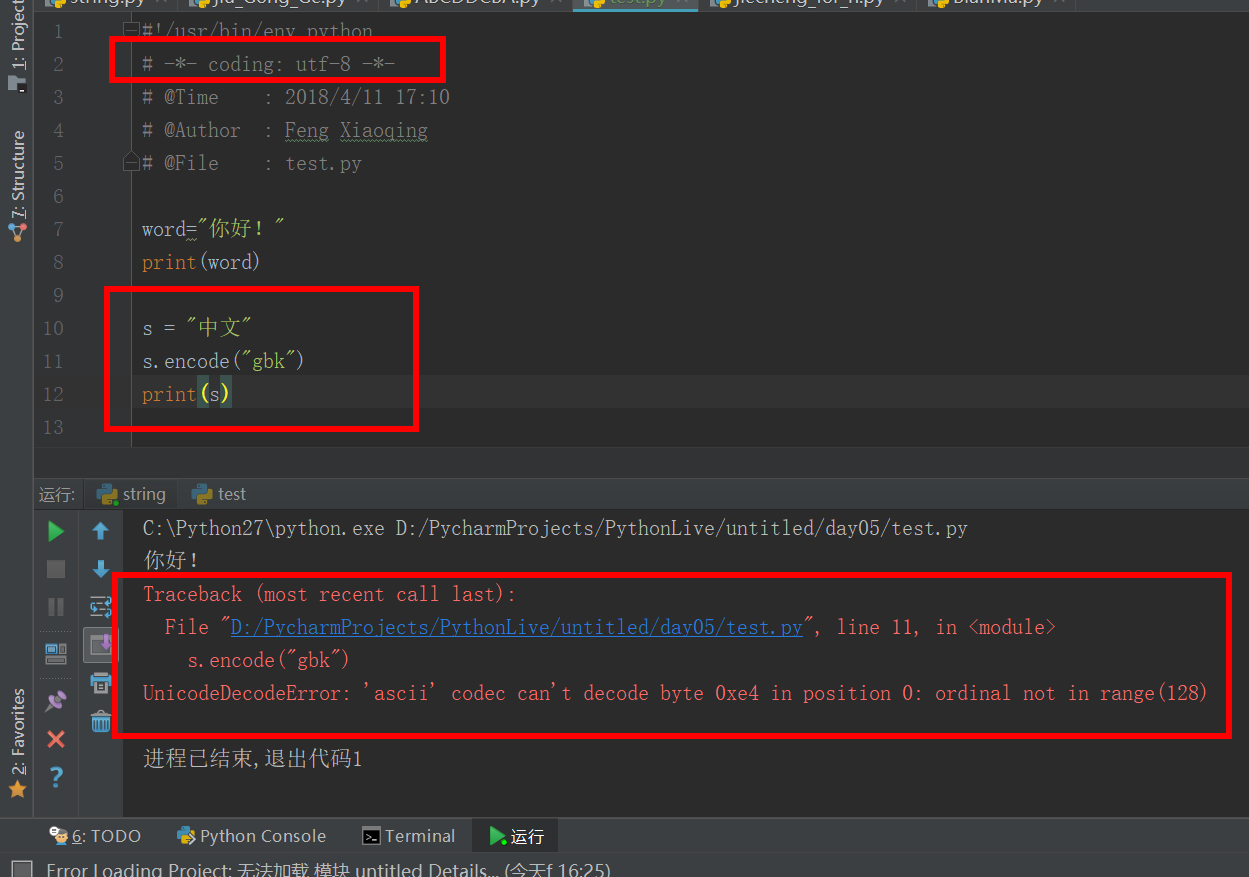
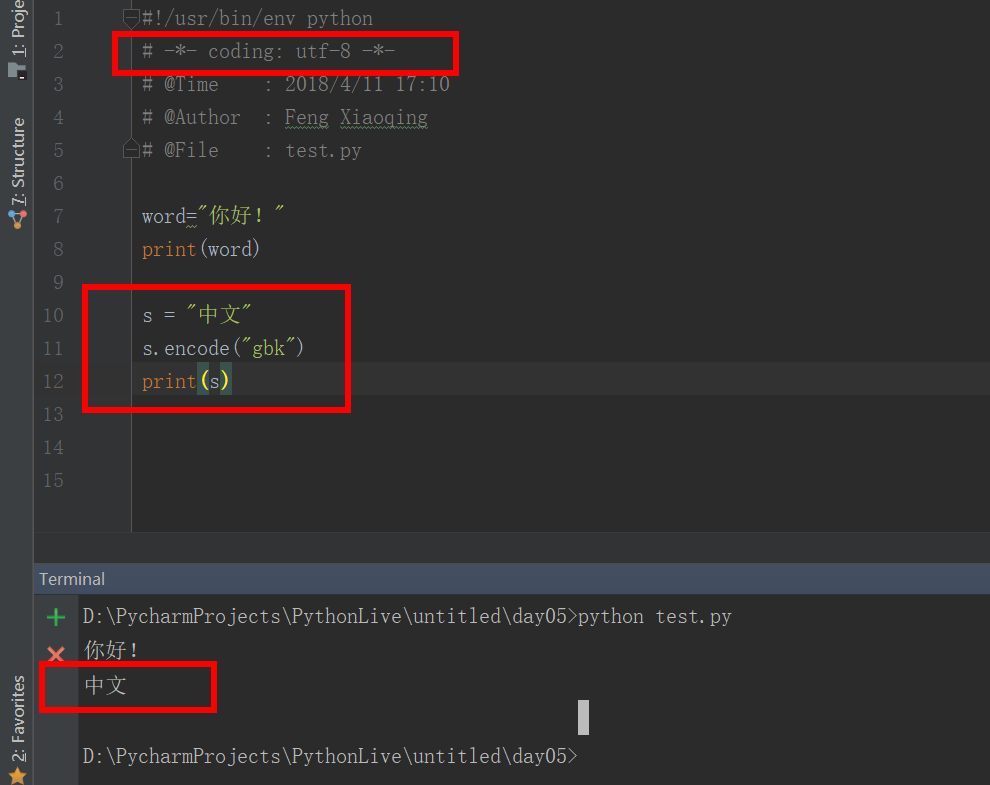
python3:
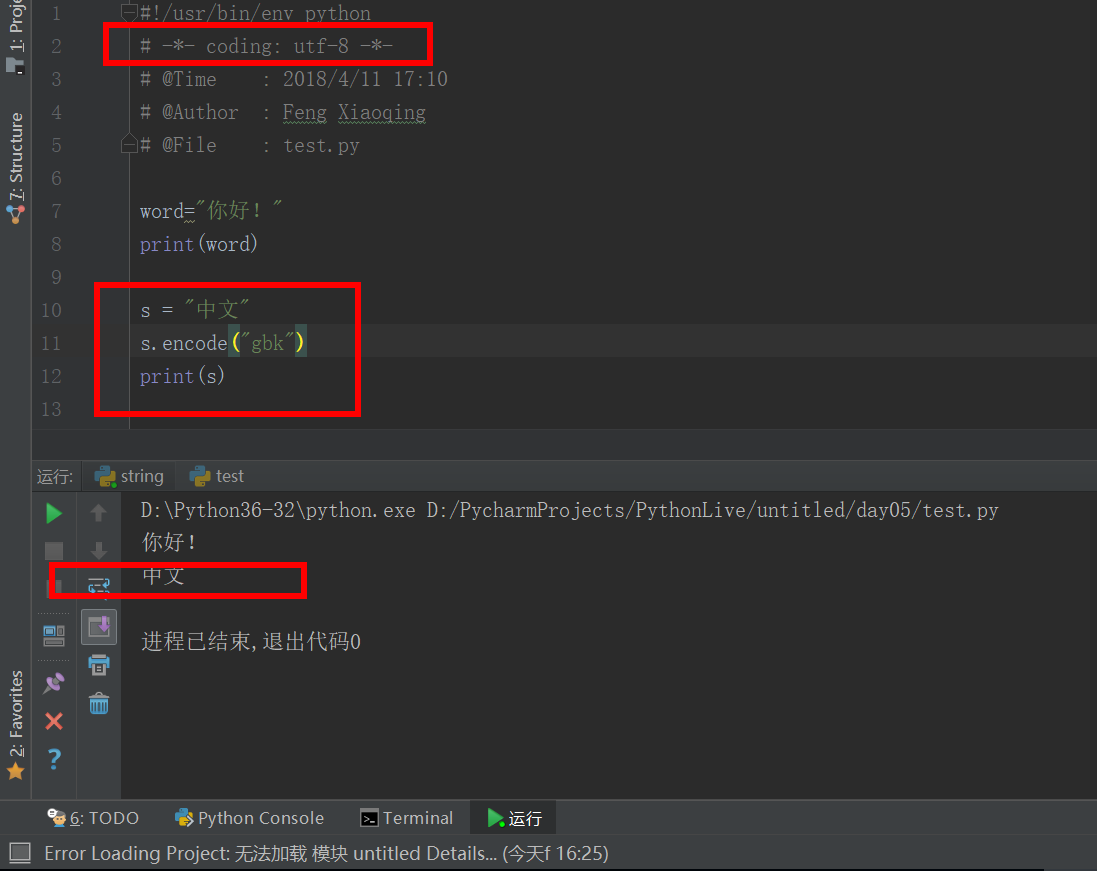